Making corpse NPCs
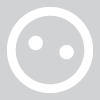
I found this tutorial which is very useful but I decided to add a twist to that:
If using an OnSpawn script on an NPC will make its corpse lie face down, and having the area do the same thing will make the corpse lie face up, is there a way to randomize that? I tried using a Random Int but as the actions are based on different events, I'm not really sure how that could be achieved. Any ideas?
If using an OnSpawn script on an NPC will make its corpse lie face down, and having the area do the same thing will make the corpse lie face up, is there a way to randomize that? I tried using a Random Int but as the actions are based on different events, I'm not really sure how that could be achieved. Any ideas?
0
Comments
I devised a system that will randomize the corpses (their position, if they're face down or up, and also add bloodstains underneath them) and it turned out great, so I thought I'd share it here for anyone who's interested:
On every area that will have corpses, go to its OnUserDefined event and add:
Create an NPC, change its tag to "Corpse" and remove all of its scripts. Then, on its OnDeath, add:
And on its OnSpawn, add:
There it is. You can change the name (e.g. "Mauled Corpse", "Strangled Woman", etc) and clothing of the corpses and give them weapons and items to create variation, as long as you keep their "Corpse" tag and use those same scripts.
TR