Custom loot - too ambitious?
Hey everyone
I am working on a PW and what I'd really like is a custom item drop system, that goes like this:
*Player kills a monster, or opens a chest* - so far it's relatively simple.
Now what I want the game to do is do some rolls to check what the player's going to grab.. to easier understand my weird thoughts, I've added a table
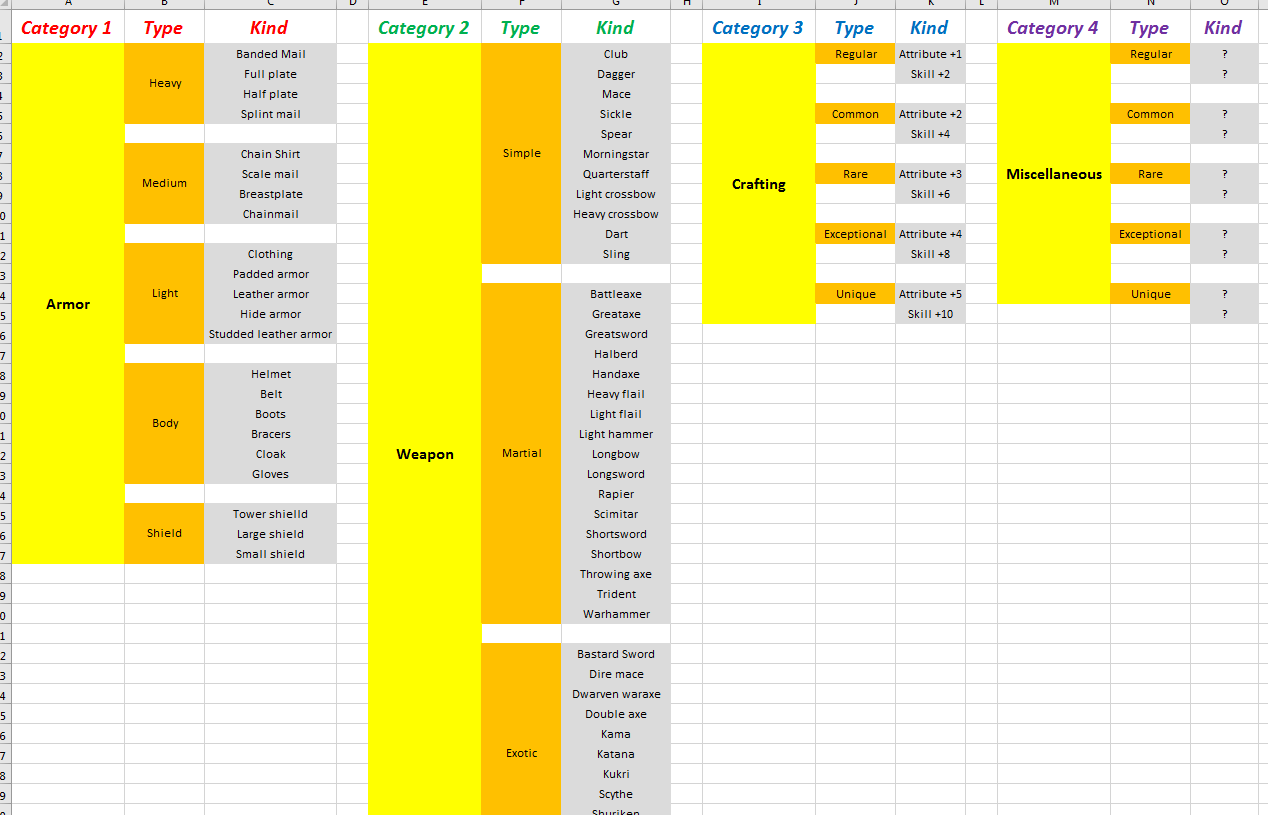
Basically, I'd like a script to roll for a category, then roll for a type of said category and finally pick the item. Since it's 4 categories, the game should roll for categories at a 25% chance to pick from 1, 2, 3 or 4. Then, depending on types inside a category - e.g. armor - roll for 5 different types at a 20% chance and finally pick an item.
There's a bit of an anomaly, since I'd like the game - should it roll for category 3 or 4 - to have the chances be distributed differently than for categories 1 and 2. Regular should have a high chance to drop, common a bit less, rare .. well.. should be rare, expectional should be exceptionally rare and unique should almost never drop.
How do I approach this? Maybe someone has an idea.. or can already tell this ain't going to work^^
I am working on a PW and what I'd really like is a custom item drop system, that goes like this:
*Player kills a monster, or opens a chest* - so far it's relatively simple.
Now what I want the game to do is do some rolls to check what the player's going to grab.. to easier understand my weird thoughts, I've added a table
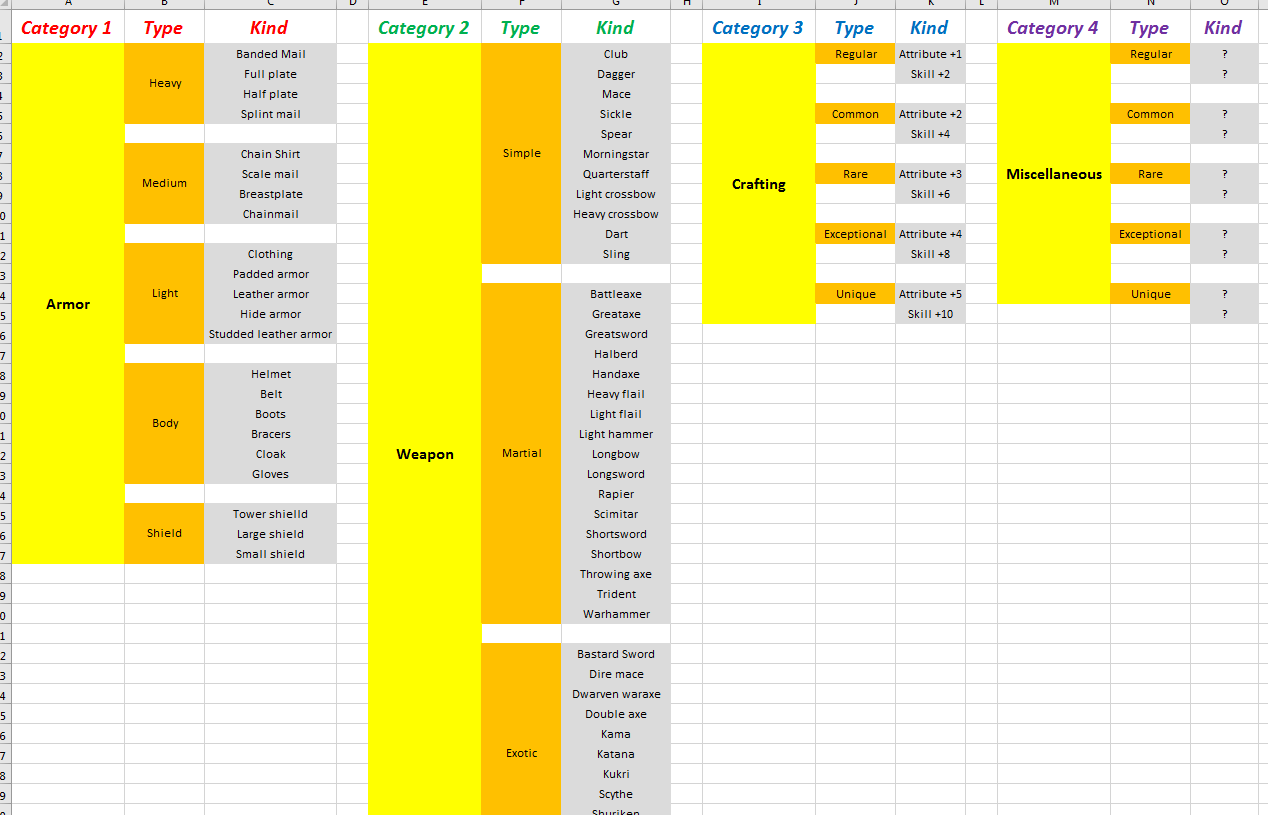
Basically, I'd like a script to roll for a category, then roll for a type of said category and finally pick the item. Since it's 4 categories, the game should roll for categories at a 25% chance to pick from 1, 2, 3 or 4. Then, depending on types inside a category - e.g. armor - roll for 5 different types at a 20% chance and finally pick an item.
There's a bit of an anomaly, since I'd like the game - should it roll for category 3 or 4 - to have the chances be distributed differently than for categories 1 and 2. Regular should have a high chance to drop, common a bit less, rare .. well.. should be rare, expectional should be exceptionally rare and unique should almost never drop.
How do I approach this? Maybe someone has an idea.. or can already tell this ain't going to work^^
1
Comments
There are a lot of different approaches you can take to do this via scripting. This is just one example I slapped together.
Thank you very much - although this looks very alien to me (I can reliably use the script wizard) I am definitely going to wrap my head around this work of you and try to make it work!
Awesome community!
Good catch. Shoulda been 94. And I skipped the 84 to 94 section. Just kind of a rough draft example I typed up real quick.
Script:
2DA file 'myitemdrop':
So how does it work: our script starts at row 0. It reads the column COUNT (= number of columns in that row) and rolls a random between 0 and that number-1. Than it looks at the value in that column and that value will be our new row. Let's say the random was 0 then our new row is 1 (category 'armor'). Again the script looks at column COUNT, rolls a random and gets a new row (something between 10 and 14). This goes on and on until the script is in a row with resrefs. Then it just returns the resref.
For chances distributed differently look at row 3 (Crafting) and row 4 (Misc): item types with higher probability appear more often.
Might be some work to build that 2da file but once it's done it should be easy to maintain. You could even use diffeerent 2da files for different classes, levels,...
The 2da cache size can be changed in settings.tml (in case you want to read many 2da files):