Follow + Random Walk
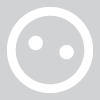
Can I have a creature walking randomly nearby the PC but start following him as soon as the PC starts moving or gets distant? And then if the PC stops the creature goes back to walking randomly? I've been trying to accomplish this using Heartbeat scripts but it's not really working. Any ideas?
0
Comments
You should check the position of the PC, with a saved position from him. If it has changed, clear all actions and follow/move to the new position, or if not walk randomly. At last, redo this check and action.
Yet, I'm not at the toolset, so I have no script handy...
The creature in question is a Lantern Archon that will follow the PC around to provide light. My next step is creating my own RandomWalk function to have more control, as I'm willing to incorporate this system to the henchman in my module.