Item Container Inventory Scripting Help
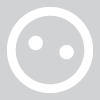
I am looking for a script function like getitemparent() or isitemincontainer(). Specifically with some of my scripts when I am doing inventory loops I want to exclude all items that are contained within container objects.
Here is a code example of where I would like to know if an individual item is in a container or not.
void TakeAllItemsLike(object oCreature, string sMatch)
{
object oItem = GetFirstItemInInventory(oCreature);
string sTAG = GetTag(oItem);
while (GetIsObjectValid(oItem))
{
sTAG = GetTag(oItem);
if (TestStringAgainstPattern("**"+sMatch+"**", GetStringUpperCase(sTAG)))
{
//Would like to check and only do this if the item is not in a specific container
DestroyObject(oItem);
}
oItem = GetNextItemInInventory(oCreature);
}
}
Thanks for any assistance.
Here is a code example of where I would like to know if an individual item is in a container or not.
void TakeAllItemsLike(object oCreature, string sMatch)
{
object oItem = GetFirstItemInInventory(oCreature);
string sTAG = GetTag(oItem);
while (GetIsObjectValid(oItem))
{
sTAG = GetTag(oItem);
if (TestStringAgainstPattern("**"+sMatch+"**", GetStringUpperCase(sTAG)))
{
//Would like to check and only do this if the item is not in a specific container
DestroyObject(oItem);
}
oItem = GetNextItemInInventory(oCreature);
}
}
Thanks for any assistance.
0
Comments
Then GetIsItemInInventory(oItem, iContainer) would return TRUE if oItem is in oContainer.
The problem however is that this function would have to use GetFirst/NextItemInInventory() and I think you can't nest GetFirst/NextItemInInventory() calls. So you can't use that function unfortunately in a GetFirst/NextItemInInventoty() loop.
Instead you would have to cycle through the items in your container first and write them down somewhere. Maybe in an array. Unfortunately while there are SetLocalArrayInt() / GetLocalArrayInt() and SetLocalArrayString() / GetLocalArrayString() functions already defined (in "nw_o0_itemmaker") there are no SetLocalArrayObject() / GetLocalArrayObject() functions. You would have to do that yourself (it's easy however, look at "nw_o0_itemmaker" to see how Bioware did it for the Int/String versions):
Then write a function SearchLocalArrayObject() that performs a search for an object in your object array:
This could be a little bit improved by automatically storing the array's size somewhere so you don't have to keep track of it yourself. I leave that up to you
Store the inventory of a container with
and to check whether an item oItem is in a container oContainer you could use:
Hope it helps.
I haven't tried to compile this, but it should be a good start.
Alrighty: The most helpful thing I can find is from here in the lexicon: "When using ActionTakeItem on a container within a container the game will crash. Suggested workaround is to place the container on the floor (preferably in the top right corner where it cannot be seen) and then perform ActionTakeItem on it."
I don't see where it suggests how to go about doing that, but if you can take the safe container out of the inventory before deleting matched items, moving it back in after should be just as easy.
With build 8193.31 and the new json datatype it's now possible to write something like getitemparent() or isitemincontainer():
https://neverwintervault.org/project/nwnee/script/scripting-functions-using-json-datatype
If you're still interested you can find among other functions
// returns TRUE if oItem in the specified container
// or in any container if no container is specified
int MK_GetIsItemInContainer(object oItem, object oContainer=OBJECT_INVALID)
and
// returns the container that holds oItem or
// OBJECT_INVALID if oItem is not in a container
object MK_GetContainer(object oItem)
in the provided include file that should do what you're looking for. Both functions do not use GetFirst/NextItemInInventory() so you can use them in GetFirst/NextItemInInventory() loops.