Help with Teleport Stuff
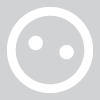
Goal #1: Have PC exchange an amulet for a map.
Goal #2: PC uses the map to teleport to a particular location.
Here are the issues I'm having:
1.) When the PC gets another map they automatically teleport to the location. It doesn't offer the option to use the item on the PC, it just teleports the PC automatically.
2.) If the PC turns in more than one amulet, the NPC gives the PC a map for each of the amulets taken.
3.) I'd like only one map to only appear in the PCs inventory - when the amulet is turned into the NPC. Then have that single map stay in the PCs inventory forever; non-droppable or tradeable.
//Put this on action taken in the conversation editor void main() { object oPC = GetPCSpeaker(); object oItem; oItem = GetFirstItemInInventory(oPC); while (GetIsObjectValid(oItem)) { if (GetTag(oItem)=="BSCULT_MARK_001") DestroyObject(oItem); oItem = GetNextItemInInventory(oPC); } CreateItemOnObject("bscult_map_001", oPC); }
Goal #2: PC uses the map to teleport to a particular location.
// ** This script uses "tag-based" item scripting. // ** If you use the item this script it named after, target yourself with the curser // then, it will teleport you to the TAG of the Waypoint named in this script. // To use: Name this SCRIPT after the ITEM to be used. On the ITEM, give it the item property "Unique Power". // Select yourself as the target of the "Unique Power"; the VFX will fire & you'll be teleported there. // // void main() { object oPC; if (!GetIsPC(GetItemActivatedTarget()) ){ SendMessageToPC(GetItemActivator(), "Improper use of item!"); return;} oPC = GetItemActivator(); object oTarget; location lTarget; oTarget = GetWaypointByTag("HELMITE_TEMPLE_001"); lTarget = GetLocation(oTarget); //only do the jump if the location is valid. //though not flawless, we just check if it is in a valid area. //the script will stop if the location isn't valid - meaning that //nothing put after the teleport will fire either. //the current location won't be stored, either if (GetAreaFromLocation(lTarget)==OBJECT_INVALID) return; AssignCommand(oPC, ClearAllActions()); DelayCommand(3.0, AssignCommand(oPC, ActionJumpToLocation(lTarget))); oTarget = oPC; //Visual effects can't be applied to waypoints, so if it is a WP //the VFX will be applied to the WP's location instead int nInt; nInt = GetObjectType(oTarget); if (nInt != OBJECT_TYPE_WAYPOINT) ApplyEffectToObject(DURATION_TYPE_INSTANT, EffectVisualEffect(VFX_IMP_UNSUMMON), oTarget); else ApplyEffectAtLocation(DURATION_TYPE_INSTANT, EffectVisualEffect(VFX_IMP_UNSUMMON), GetLocation(oTarget)); }
Here are the issues I'm having:
1.) When the PC gets another map they automatically teleport to the location. It doesn't offer the option to use the item on the PC, it just teleports the PC automatically.
2.) If the PC turns in more than one amulet, the NPC gives the PC a map for each of the amulets taken.
3.) I'd like only one map to only appear in the PCs inventory - when the amulet is turned into the NPC. Then have that single map stay in the PCs inventory forever; non-droppable or tradeable.
0
Comments
To avoid creating more than one map, SetLocalInt on the PC when the map is first given. At the start of the script, if GetLocalInt is set on the PC, return without doing anything.
The problem with Goal #2 is that tag-based scripts fire for every item event, including Acquire.
You only want the script to do something on Activate.
This tutorial shows exactly how:
https://nwnlexicon.com/index.php/Grimlar_-_Introduction_To_Tag_Based_Scripting
Then there's all the tutorials linked to in this pinned thread on the vault -
The Starting Point - module building tutorials for beginners - and where to find them
Then there's the Scripting Frequently Asked Questions (with answers - includes tutorials) -
Just The FAQs, ma'am
And the tutorials that I wrote. Start with -
TR's Basics - Variables, Types and Functions (TBC)
Finally there are all the prefab items and systems linked to in this pinned thread on the vault that require little to no scripting -
Books & Things
TR
The script you posted with the check added:
This is basically the same script but cleaned up a bit:
Edit: 2nd script had an extra set of curly brackets left over. I removed them.
TR