CopyObject() used on traps results in a trigger object that cannot be detected by players
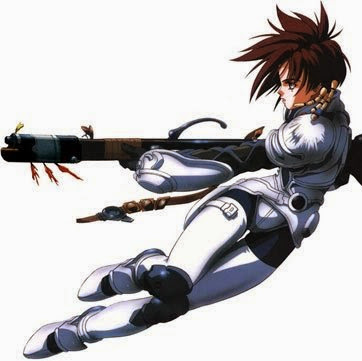
When I use CopyObject() on a trap-type trigger the resulting trap is normal (it can be triggered, etc) except that it cannot be detected. It can be force detected with SetTrapDetectedBy() but the Search skill seems to be utterly useless. Is this a bug? Is there any way to get around this? Can this be fixed?
0
Comments
As for CopyObject, which object is running the script? Did you set the owner and copy variables parameters? It sounds like a bug, but I just wonder... for example, if the module is implicitly setting the trap, or something like that...
I've been going round and round with this problem for days and peeling apart every bit of code I can to make absolutely sure it's a flaw with the game and not my code and all I can conclude is that it's something with the CopyObject() function that is not working either as intended or as I would expect. All I can think of is that the copied traps aren't getting populated into some list that Search is looking at or there's something improper about the way they are created that makes them not interact with that skill.
Each area uses the same OnEnter script to dynamically spawn in NPCs and such when the first player enters and an OnExit script despawns the NPCs (but leaves the placeables and other things without heartbeats) when the last player leaves. Among the stuff that this script is doing is the following subroutine for traps, executed as it iterates through every object in the area (oObject):
Here you can see I execute the StoreTrapFromObject() function (which I'll detail below), saving all the properties of the trap onto it in variable form, then I neuter the trap. I don't want to delete it because when it is copied the copy will share its shape. Then I can reactivate the cloned version of the trap.
Next, the script checks if the trap currently has a clone out there (so that we don't create another clone every time a player first enters). If there's no clone, then we spawn a new clone just like the original trap, otherwise we leave it be.
Here are the called functions:
Store every collectable property of a trap in variable form for later recovery and mark it so that you only do this once. This "parent" trap will only be used as a reference to clone "child" traps from, copying the parent's shape and then restoring all the relevant properties from.
This function retrieves all the properties from the "parent" trap, creates a clone, makes the parent and child aware of each other and marks the child as a clone, then assigns all the saved properties to the child. It's important to note that SetTrapDetectable() works just fine here. The child trap is correctly flagged as detectable and this is provable by interrogating the child trap with a script. It's just that the Search skill fails to find it, ever. This is why I believe it's a bug.
Lastly is just a function to identify a valid deactivated trap from the soft-delete removal that happens to traps which have been sprung (they can still be found by reference, but their positions are set to a null vector and they lose all properties, it's weird).