Setting a local var for 60 seconds?
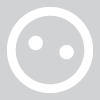
I want to set a local var and delete it after 60 seconds. DelayCommand doesn't work on DeleteLocalInt. Any ideas on how to do this?
Basically, I have a special potion. The player drinks it and for 60 seconds they have double XP rewards. If they drink it again, it should reset the timer.
In my XP script I can check for if a LocalInt is set, but not sure how to get it deleted after 60 seconds unless I want to get into a bunch of calendar math. Is there a simple way to do this ?
I could deincrement on heart beat script, but wondering if there is any other ways?
Basically, I have a special potion. The player drinks it and for 60 seconds they have double XP rewards. If they drink it again, it should reset the timer.
In my XP script I can check for if a LocalInt is set, but not sure how to get it deleted after 60 seconds unless I want to get into a bunch of calendar math. Is there a simple way to do this ?
I could deincrement on heart beat script, but wondering if there is any other ways?
0
Comments
In the meantime, here's a sample function that does what you need it to.
void SetTemporaryInt(object oTarget, string sVarName, int nValue, float fDelay) { SetLocalInt(oTarget, sVarName, nValue); DelayCommand(fDelay, DeleteLocalInt(oTarget, sVarName)); }
EDIT: D'oh! Forgot that you wanted to refresh the duration of the potion.
The only way is to kill the calling object
I guess with some workarround you could make sure to eliminate previous DelayCommand() calls.
Something like (not tested):
void DeleteTemporaryInt(object oTarget, string sVarName, int iScriptID) { if (iScriptID == GetLocalInt(oTarget, "CURRENT_SCRIPT_ID")) DeleteLocalInt(oTarget, sVarName); } void main() { object oTarget = OBJECT_SELF; string sVarName = "XP_POTION"; SetLocalInt(oTarget , "CURRENT_SCRIPT_ID", GetLocalInt(oTarget , "CURRENT_SCRIPT_ID") + 1); int iScriptID = GetLocalInt(oTarget , "CURRENT_SCRIPT_ID"); SetLocalInt(oTarget, sVarName, 1); DelayCommand(60.0, DeleteTemporaryInt(oTarget, sVarName, iScriptID)); }
Basically the CURRENT_SCRIPT_ID local var just makes sure to always only execute the latest DelayCommand call.
If you worry about an overflow you can always reset CURRENT_SCRIPT_ID whenever it reaches 2,147,483,647
00-20: 1
20-40: 2
40-60: 3
60-80: 2
80-100: 1
120+: 0
So then your code would look something like
if (GetLocalInt(OBJECT_SELF, "doublexp")) then give double xp
Above example is much simpler.
No need to fuzzle arround with deleting variables.
Gives you more control than DelayCommand, which is fire-and-forget.
I can script it, but hoping there is easy way to attach on effect so can work like that. (or make a simple effect with custom var or something).
The problem:
Player drinks potion, set var =1
DelayCommand1 (deletevar, 60 )
At 30 seconds Player sleeps, deletevar
wakes up, Drinks new potion
DelayCommand 2(deletevar, 60 )
Delaycommand1 deletes it after 30 seconds
Dave
If you've never done this before, I'd start with reading the Spells section of Eligio's Custom Content Guide:
https://neverwintervault.org/project/nwn1/other/custom-content-guide-v30
There are some wrinkles you may bump into, but you can make this work. Give it a shot, see what you can figure out with Eligio's write up, and feel free to follow up with any questions.
-Dave