Infinite Loop - Debug Help
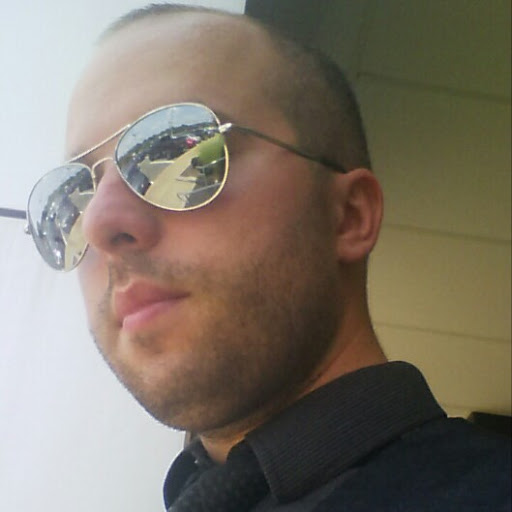
I don't know if I'm just a moron or if I've been staring at this code for too long. Possibly both. I can't for the life of me figure out why this function is creating a persistent loop.
For some reason my code is grabbing the first object in the area over and over and over, creating hundreds of the placeable on the first object's location. and causing too many instructions. I was able to determine via debug text that the GetNextObjectInArea() seems to not be firing, because GetIsObjectValid() remains true hundreds of times despite there only being 10 objects in the area.
I'm wondering if there is some nuance or bug with the CreateObject call, I've had many issues with the function in the past causing unreliable results when called directly and not nested in a separate function.
void SpawnTrialObjects(object oArea) { string sTag; location lPlaceable; object oWP = GetFirstObjectInArea(oArea); while (GetIsObjectValid(oWP)) { sTag = GetTag(oWP); SendMessageToPC(GetFirstPC(), "Fired on name: " + GetName(oWP)); if (sTag == "dgn_buff1" || sTag == "dgn_buff2" || sTag == "dgn_buff3" || sTag == "dgn_next" || sTag == "dgn_exit") { sTag = "p_" + sTag; lPlaceable = GetLocation(oWP); ApplyEffectAtLocation(DURATION_TYPE_INSTANT, EffectVisualEffect(VFX_IMP_PULSE_WIND), lPlaceable); CreateObject(OBJECT_TYPE_PLACEABLE, sTag, lPlaceable); } oWP = GetNextObjectInArea(oArea); } }Basically, this function loops through an area checking each object (the area has 10 objects, nothing major) and will create appropriate placeable objects at the locations of hidden waypoints (for instancing reasons I can't use GetWaypointByTag())
For some reason my code is grabbing the first object in the area over and over and over, creating hundreds of the placeable on the first object's location. and causing too many instructions. I was able to determine via debug text that the GetNextObjectInArea() seems to not be firing, because GetIsObjectValid() remains true hundreds of times despite there only being 10 objects in the area.
I'm wondering if there is some nuance or bug with the CreateObject call, I've had many issues with the function in the past causing unreliable results when called directly and not nested in a separate function.
0
Comments
The below code works perfectly
By introducing a delay in the second case, you are only scanning the original objects, not the new ones.