Get Position within trigger?
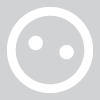
I'm trying to develop a system that will spawn monsters at positions close to the walls of a room. Instead of using several waypoints, I wondered if it would be possible to use a big trigger and find random positions within it and then, close to its borders. Is there anything similar to "GetPositionWithinTrigger" or a possible workaround?
0
Comments
The simplest method is to use CreateObject to spawn the monster at the computed location. You can also spawn waypoints if you need them for some reason.
Since you know the coordinates of the room in advance, you can use the Random function to choose a random wall, then a random x and random y close to that wall.
A refinement is to place reference waypoints at two diagonally opposite corners, then discover the room coordinates via GetLocation. That way, the script will still work if the area is resized.
The Random function can also be used to create a "random pair algorithm" if you need it.