AI scripting examples
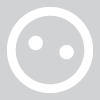
Would anyone who has done AI scripting successfully care to post some examples/explanations for us to learn from? I've not seen specific AI scripting examples discussed in much detail here and think there's quite a bit of interest in making interesting monster behavior.
I've tried a lot of stuff with inconsistent success at best. (at least I have fun trying). I've looked at the x2_ai_... options but clearly don't appreciate everything that is being done in those and why.
I've tried a lot of stuff with inconsistent success at best. (at least I have fun trying). I've looked at the x2_ai_... options but clearly don't appreciate everything that is being done in those and why.
0
Comments
1. This is my basic AI script for my Darkener NPC to ensure that it casts darkness when I want (or try to dispel UV/true seeing if they have that up). I've put some comments about parts I don't understand/appreciate what they do. Comments/explanations are welcome.
2. Share a script to show off what you did. (Maybe AI scripts are inherently too long and complicated for this format, I dunno.)
in the hakpack. all the ai scripts are charactername+ai. although they won't function without all the other scripts, etc.
and. the char ai script... it's long, and broken lol.