I think I messed up a script using the "DoOnce" command.
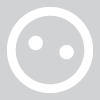
I'm trying to give the PC a Recall Stone & some gold the first time they log into the persistent world. The first time they log in, it will be in a starting area. Then, every time after their first log-in into the PW, they don't get the Recall Stone & gold again. This is what I have so far - I thought the DoOnce command would solve this issue, but I was wrong.
// ** Gives a Stone of Recall to player on entering this area
//Put this script OnEnter
//** #include "nw_i0_tool" is for giving gold to player on enter of starting area.
#include "nw_i0_tool"
void main()
{
object oPC = GetEnteringObject();
if (!GetIsPC(oPC)) return;
int DoOnce = GetLocalInt(oPC, GetTag(OBJECT_SELF));
if (DoOnce==TRUE) return;
SetLocalInt(oPC, GetTag(OBJECT_SELF), TRUE);
CreateItemOnObject("nw_it_recall", oPC);
RewardPartyGP(50, oPC, TRUE);
}
// ** Gives a Stone of Recall to player on entering this area
//Put this script OnEnter
//** #include "nw_i0_tool" is for giving gold to player on enter of starting area.
#include "nw_i0_tool"
void main()
{
object oPC = GetEnteringObject();
if (!GetIsPC(oPC)) return;
int DoOnce = GetLocalInt(oPC, GetTag(OBJECT_SELF));
if (DoOnce==TRUE) return;
SetLocalInt(oPC, GetTag(OBJECT_SELF), TRUE);
CreateItemOnObject("nw_it_recall", oPC);
RewardPartyGP(50, oPC, TRUE);
}
0
Comments
Variables set on objects in a PC's inventory do carry over. You can give your player an undroppable item that you use to store variables for the PC across resets. Otherwise, you'll need to use a database.
Alternatively, just check if they have one. If not, give them one.
// ** Gives a Stone of Recall to player on entering this area
//Put this script OnEnter
//** #include "nw_i0_tool" is for giving gold to player on enter of starting area.
#include "nw_i0_tool"
void main()
{
object oPC = GetEnteringObject();
if (!GetIsPC(oPC)) return;
int DoOnce = GetLocalInt(oPC, GetTag(OBJECT_SELF));
if (DoOnce==TRUE) return;
if( GetItemPossessedBy(oPC,"NW_IT_RECALL") == OBJECT_INVALID ) // Making recall stone only drop once.
SetLocalInt(oPC, GetTag(OBJECT_SELF), TRUE);
CreateItemOnObject("nw_it_recall", oPC);
RewardPartyGP(50, oPC, TRUE);
}
Also, when I try to test in in multiplayer, sometimes it crashes the game when selecting a saved character. More help and ideas are welcome!
Are you putting this in the area OnEnter or the module OnClientEnter?
You did forget to put brackets on the last if statement. That can make it do unexpected things. Actually, you didn't put brackets on any of the if statements. That can lead to many painful hours scouring for what could be wrong.
Anyway, you don't need the DoOnce part anymore. It wouldn't have worked anyway unless you have a player tagging system that gives players a unique tag when they enter the module. (I've shared how to do that on this forum before elsewhere.)
Here's the revised script:
If you do want to put it in the module level OnClientEnter, I recommend just making it a new script and adding it to the OnClientEnter script with ExecuteScript (I named it give_recall):