rotating objects (need a bit of help)
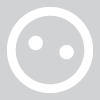
I have a few levers that I am using to spin a placeable, it is working but I am having a hard time figuring out how the float is actually working. This script spins the placeable 360 degrees which is what I want, but it only works the first time I pull the lever. I would like it to spin 360 degrees every time I pull it.
I need one that spins 90 degrees counter clockwise another 90 degrees clockwise, and one that spins 180 degrees clockwise. setting x = 90 or - 90 does provide results, just not the desired results.
I need some help!
//360 degree rotation void main() { object oPC = GetLastUsedBy(); object oTarget = GetObjectByTag(GetTag(OBJECT_SELF)+ "_Target"); float x = GetFacing(oTarget); x = 360.0; SetObjectVisualTransform(oTarget, OBJECT_VISUAL_TRANSFORM_ROTATE_X, x, OBJECT_VISUAL_TRANSFORM_LERP_LINEAR, 6.0, TRUE); PlaySound("as_cv_brickscrp1"); DelayCommand(0.50, PlaySound("as_cv_brickscrp1")); DelayCommand(1.0, PlaySound("as_cv_brickscrp1")); DelayCommand(1.5, PlaySound("as_cv_brickscrp1")); DelayCommand(2.0, PlaySound("as_cv_brickscrp1")); DelayCommand(2.5, PlaySound("as_cv_brickscrp1")); DelayCommand(3.0, PlaySound("as_cv_brickscrp1")); DelayCommand(3.5, PlaySound("as_cv_brickscrp1")); DelayCommand(4.0, PlaySound("as_cv_brickscrp1")); DelayCommand(4.5, PlaySound("as_cv_brickscrp1")); DelayCommand(5.0, PlaySound("as_cv_brickscrp1")); DelayCommand(5.5, PlaySound("as_cv_brickscrp1")); }
I need one that spins 90 degrees counter clockwise another 90 degrees clockwise, and one that spins 180 degrees clockwise. setting x = 90 or - 90 does provide results, just not the desired results.
I need some help!
0
Comments
Custom Content Challenge - January 2022 : Dual Themes (Once Upon a Time & Skyboxes).
TR
SetObjectVisualTransform can be reset by repeating the previous command with a value and time of zero. So, rotate 360, wait, rotate 0 then immediately rotate 360 again.
In my tests, reset followed by transform happens so fast that you don't see the reset - which is obviously not an issue with 360 rotation in any case.
IIRC the reset is independent for each type of transform, so, for example, resetting the X rotation does not cancel a Z translation.
It seems to just jump to the new position.
I am trying to have the Stone Skull head rotate in the middle of a room after a lever is pulled. This along with the grinding stone sound has a nice mechanical affect. A certain pattern of rotation will hopefully unlock a door.
Hope it helps.
If you have to use SetFacing(), use delays to rotate it in small angular steps - but that will never be as smooth.
I used NeverwinterWights script and then set some variables on the object with a small amount of math and if statements to keep track of the direction.
if the face is facing a door, the clockwise 360 spin unlocks and opens it. The counter clockwise 360 closes and locks it.
Only one door can be open at a time.
Fairly simple puzzle really, but cool action in game.