Can I get ID of currently active character?
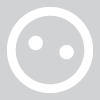
in UI Modding
Hello,
I'd been working on my modified Stealth ability, but was never satisfied with the fact, that it was a Special Ability, and not something you could trigger via Stealth button from the main Actionbar. Today I've found a potential solution to my problem, that doesn't even require EEEx, however due to my lack of experience with UI modding, I am not sure how to finalize my implementation.
I can overwrite the original action from
Do you know if such index is possible to get instantly, or if it can be somehow mapped from some other data?
PS. this CHARACTER_ID is an optional parameter, but its value is NOT current character ID if I don't provide it at all, so getting rid of it is not the solution.
Thank you very much for your help!
I'd been working on my modified Stealth ability, but was never satisfied with the fact, that it was a Special Ability, and not something you could trigger via Stealth button from the main Actionbar. Today I've found a potential solution to my problem, that doesn't even require EEEx, however due to my lack of experience with UI modding, I am not sure how to finalize my implementation.
I can overwrite the original action from
action "buttonArray:OnLButtonPressed(X)"to
action "C:Eval('ReallyForceSpell(Myself,MY_STEALTH)', CHARACTER_ID)"and it works well, but I need a way to dynamically pass that "CHARACTER_ID" value, which IESDP describes as:
CHARACTER_ID is an optional numeric parameter specifies the active party member for the script action. The parameter expects the portrait slot index, starting with 0 for the first party member.
Do you know if such index is possible to get instantly, or if it can be somehow mapped from some other data?
PS. this CHARACTER_ID is an optional parameter, but its value is NOT current character ID if I don't provide it at all, so getting rid of it is not the solution.
Thank you very much for your help!
0
Comments
First, you can use or to get the name of the selected character.
Second, you can use (i = 0~5, being the CHARACTER_ID) to get the PortraitTooltip of a specific character. The PortraitTooltip is like this: I think we're pretty close.
You came to the same solution I had at some point. However thanks to @Pecca I managed to get what I think is a better and more reliable solution, although far more complicated.
Here's my main function: This ensures that we get the proper ID, no matter if there are characters with the same name for example. But the problem here is that this code actually clicks through all portraits, which makes the characters say their standard selection phrases. To avoid that I've implemented functions that deactivate those selection sounds and bring them back to the original state right after the selection is finished:
And finally here's the usage of this code in practice:
Yes, your method is definitely better. However I don't understand how does the C:Eval part works. If I get it right, charPortraitId is a number 0~5, so the C:Eval part should be like: How does this work? I tested several commands like this using the console and nothing happened.
This works well. I have to warn you though - using Stealth from F5 (or any other) keyboard shortcut will still trigger standard Stealth behavior.
Now I have another problem: how do I type "SPCLXXX" into this code? It seems that the program recognize anything inside "" as some token. I tried [""] and \"\", didn't work.
Okay, here comes another another problem. I've been trying to create my own Hide in Plain Sight ability. So far the best approach I come up with is to apply a spell that temporarily sets the character's visual range to 0 before stealth. It worked somehow, but it didn't work well.
If I do this by after I press the Stealth button it becomes gray immediately and the character stops stealth.
Or I can do this by Everything seems right after I press the button. HOWEVER I can press the button anytime, even when it's gray, and it still functions.
What's your design of the spell? It seems quite complicated to make such a spell. I can think of a way to check the Stealth points (stats 27 I think), maybe day/night and indoor/outside too, but I don't know how to check whether the character stands in light or shadow.
If you just want to have Hide in Plain Sight and use standard Hide() action, then I am sure it can be done rather easily, though preventing players from clicking the button until they get their desired result is a bit more complicated, it would require giving your spell that blinds you something else, that could be accessed through UI. Firstly, you need to change to So the action function should look something like that: As from my observations buttonArray:OnLButtonPressed(5) action is fired before the spell from Eval.
And secondly, you need to make sure that your spell, which blinds your character:
1. Targets self
2. Is non-hostile and doesn't break sanctuary/invisibility (flags at offset 0x18)
3. Applies blind only for 1 game tick (using timing mode=10)
That way the blindness shouldn't be problematic and you should get proper order of actions, firstly the spell and right after that HiS attempt. Here's the result (the planetar is hostile). And ofc it would be better to create a spell that has no name and only applies blind, to not clutter the log.
I found a way to make sure that players can only trigger sneak once per round. I used Infinity_GetClockTicks() to time the time between two clicks.