Conditions for Conversations
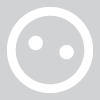
fingers crossed,
After puzzling for an entire evening over getting conditions working for conversations, thought I would share at least one script that appears to work in the game without fault. This will hopefully save you a lot of time messing around trying to get it working correctly.
This one is a check for nFirstTimeTalked, one of the most useful conditions to have in a conversation. This is when the player is speaking to the NPC (or whatever it is they are talking to) for the first time.
int StartingConditional()
{
// Inspect local variables
if(!(GetLocalInt(GetPCSpeaker(), "nFirstTimeTalked") == 0))
return FALSE;
SetLocalInt(GetPCSpeaker(), "nFirstTimeTalked", 1);
return TRUE;
}
Still trying to figure out how to get other conditions working as it seems that the Script Wizard in the conversation editor does not produce reliable scripts - at least from my experience.
After puzzling for an entire evening over getting conditions working for conversations, thought I would share at least one script that appears to work in the game without fault. This will hopefully save you a lot of time messing around trying to get it working correctly.
This one is a check for nFirstTimeTalked, one of the most useful conditions to have in a conversation. This is when the player is speaking to the NPC (or whatever it is they are talking to) for the first time.
int StartingConditional()
{
// Inspect local variables
if(!(GetLocalInt(GetPCSpeaker(), "nFirstTimeTalked") == 0))
return FALSE;
SetLocalInt(GetPCSpeaker(), "nFirstTimeTalked", 1);
return TRUE;
}
Still trying to figure out how to get other conditions working as it seems that the Script Wizard in the conversation editor does not produce reliable scripts - at least from my experience.
Post edited by nwfan87 on
0
Comments
TR
It takes five commands, but that's just because I used two "setup" lines for readability.
Tried using the script and low and behold, still getting the same behavior, the NPC will not speak the other dialogue tree at all, its triggering the opposite dialogue tree to what I'm hoping for. p.s I gave up on the FirstTimeTalked as it was just too much. Just using Journal checks for now.
If it doesn't work, my first guess is that you might have the logic mixed up. Think of it like this: If a NPC line has a conditional and it returns TRUE for any reason, then that line is selected for the conversation and any later lines do not run. if it returns FALSE then that line is not selected and it tries the conditional for the next line. Make sure that the last line does not have a conditional and is the line which should run if none of the conditions have been met.
In this case, the first conversation line should be the "we've spoken before" line, while the second (and last) line should be the "we've never spoken before" line.
Another guess would be that you somehow made the NPC de-spawn, or he's being killed. If either is the case, then when he respawns he won't have any of the variables which were set on his previous instance. The easiest way of handling this would be to use the PC (in single player) or an undroppable item on the PC (in multiplayer) to save the variables to.
Single-player version:
Multiplayer version (assuming the PC is carrying an item tagged "ToolBox"):
(Oh, and it's "lo" short for "hello" which was once an expression of surprise, not a greeting; hence "lo and behold" is a short but deliberately grandiose way of saying "this is surprising, look here")
Now I'm to the point of wishing I could see the conversation so that I could help you to figure out the problem and fix it, but I hope that the information I've given here helps.
Thing deteriorated even more to the point where the scripts won't compile, so now relying on triggers, which isn't ideal and causes problems.
It would be good to have scripts (before have too much to change) that:
journals checks,
int checks
item checks
As a question, do you need checks on every starting NPC line in a conversation file? NPC's are always starts with the non conditional option, rather than the ones which have checks.
Here are the scripts that I've tried to use.
In advance, thank you so much for your help!!
journal check
int StartingConditional()
{
if (GetLocalInt(GetPCSpeaker, "NW_JOURNAL_ENTRYratkiller")==2);
}
int check
int StartingConditional()
{
// Inspect local variables
if(!(GetLocalInt(GetPCSpeaker(), "has_spoken_to_the_zombie") == 1))
return FALSE;
return TRUE;
}
item check
#include "nw_i0_tool"
int StartingConditional()
{
// Make sure the PC speaker has these items in their inventory
if(!HasItem(GetPCSpeaker(), "rat_tail"))
return FALSE;
return TRUE;
}
That first one won't compile because you don't have return statements.
It sounds like you may want to look at the scripting faq and maybe the toolset guide. I don't have links handy but Tarot linked the faq above. The lexicon is also useful. But the things you are trying to do are very common and are covered (with examples) in some of those docs I'm sure.
I will have to do some hunting around to find some good scripting things. Tarot already posted some links to some good resources.
Putting it another way, when the NPC conversation comes up, the game looks at the first conversation line and checks if there's a conditional attached. If there isn't, it selects that line. If there is, then it runs the conditional and only selects that line of conversation if the result is TRUE, otherwise it drops down to the next line of conversation at that level and does the whole thing over again. Take the following conversation for example (NPC lines have a single dash while PC lines have a double dash):
- Hello again, <name>, nice to see you again. Would you like to see my shop?
- - Yes, thanks.
- - Not this time.
- Hello, you seem to be new here, but it's nice to meet you. Would you like to buy or sell things?
- - Yes, thanks.
- - Not this time.
Here's the single-player version of the script, again: If you place the above script into the Appears When of the line "Hello again" then that should do what you want.
You don't put anything into the "Hello, you seem to be new..." line, because you want it to be selected if the "Hello again" line isn't selected.
Both the "Hello" lines are at the first level, but the first line only runs if the script returns TRUE. The second "Hello" line does not run at all if the first line is selected.
better version:
In that case, that would also reflect why it is important to put non conditional lines as the last line. If it was the first, it would only return that line, ever, regardless of any changes to conditionals?
@nwfan87 Yes, you have correctly understood how the game processes a conversation file in order to decide which line the NPC should speak.
It gets even more fun when you apply an "appears when" script to player lines. Doing this allows you to deny a player certain options (if the PC does not qualify for them, for example) by making them never appear in the conversation at all, so they can't be selected. If the conditional returns FALSE then the player conversation line does not even show up at all. Be careful that there is always at least one valid option for the player to select, though.
@Melkior That is good then, glad I got the logic right. Don't think I'll be doing any appears when options. Comes down mostly to how much I can tolerate my own lack of skills with scripting and conversations. If its taking too much time, I'll find some other solution which present less problems. Better that way than spend 100 hours working on one conversation and losing motivation, sacrificing all the work done thus far. Even with all this help, I'm still having issues, but I'll just do my best from here on out and see what works and doesn't - the joy of scripting
There is a large list of the built-in functions of that type, included with the FAQs I linked to early in this thread.
TR
@Melkior @meaglyn I managed to make some progress with the conversation files. It was just a matter of reorganizing the logic of it, which can be difficult if you have the same NPC with say, 50 starting lines or so, or, you've never made a conversation file larger than one thread. Really have to take the time, working out the order. I think I read somewhere else other than this thread, working 'backwards' in terms of where you expect the NPC to use a particular thread in a plot, helps, which it has for me.
With this, I'll hope to be able to make some more progress as I get to grips with this system.