Question about switch/case inside loop
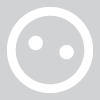
In a loop like this, shouldn't the switch/case changes in the iX and iY values happen separately? When I use this, they add up to one another (case 1, then case 1 + case 2, etc). I tried using if/elses but the same thing happened. Is there something here I'm not seeing? I'm trying to create a chess game and this script is supposed to check the availability of spaces around the king.
int xCount = 1; for (xCount = 1; xCount <= 8; xCount++) { switch (xCount) { case 1: iY++; break; /// Forward case 2: iX++; break; /// Forward + Right case 3: iY--; break; /// Right case 4: iY--; break; /// Backward + Right case 5: iX--; break; /// Backward case 6: iX--; break; /// Backward + Left case 7: iY++; break; /// Left case 8: iY++; break; /// Forward + Left }
0
Comments
A better way is to have "interim" variables which take the value of iX and iY after either adding or subtracting one, as required. This will leave the iX and iY variables unchanged so that they don't need to be re-set on each pass. You use iX1 and iY1 instead of iX and iY, so that you don't disturb the values of the original iX and iY variables.
Because you're going through the loop eight times, the instructions on every line get done once, so by the time the loop ends, the value in the variables will wind up being the sum of all of those increments and decrements.
If I manually add and subtract all of those lines, as would happen if the loop ran 8 times, then by the time the loop ends, iX will wind up being one less than it was while iY will wind up being one more than it was. If that's not what you intended, then there's the source of your problem.
In the interim, the values of iX and iY will probably wind up with them pointing to the wrong places.
(Note that when I made the above code, I assumed an array starting at the top, left rather than bottom left. You'll need to reverse the + and - for the values of iY if your code assumes starting at bottom left)