Help with circuit system
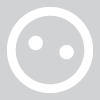
I've been trying to exercise my scripting by writing my own circuit system. It's basically a walkway system which allows random/non-random animations to be added as the creature reaches a waypoint. Everything goes in a single OnHeartBeat function and, up to now, it's been working great.
As soon as the creature starts moving from one waypoint to the other, an integer is checked so that the function won't override itself. When the movement and the animation is over, the integer resets and the next OnHB will trigger it again.
The thing is, if the creature is disturbed during the circuit, the integer will not reset and the system will just stop working. Is there simple way to replace this integer with another kind of check? Such as "is the creature moving/performing an animation"?
Any ideas are welcome.
As soon as the creature starts moving from one waypoint to the other, an integer is checked so that the function won't override itself. When the movement and the animation is over, the integer resets and the next OnHB will trigger it again.
The thing is, if the creature is disturbed during the circuit, the integer will not reset and the system will just stop working. Is there simple way to replace this integer with another kind of check? Such as "is the creature moving/performing an animation"?
Any ideas are welcome.
0
Comments
2. Heartbeat continues to run, but doesn't act on this NPC because it has the Int set.
3. NPC arrives and does some animation afterwhich the Int is reset so the NPC can accept new commands on future heartbeats.
If you just need suggestions to get the ideas flowing then I'll throw this idea out. (this is untested, but the idea is there)
Set the waypoint object the NPC should be going to on the NPC (at the same time you set the Int).
SetLocalObject(oNPC,"WP_IM_GOING_TO",GetWaypointByTag("TAG_OF_WP"));
The heartbeat could check the distance between the NPC and the waypoint and set that on the NPC if it hasn't already.
If it has, then it can monitor if the distance between the two is decreasing.
If the distance is staying the same or increasing over several iterations (and they aren't in combat), then tell them to get moving again.
[begin shameless plug] You can also checkout my advanced walkwaypoints system which expands the builtin system with among other things the same sort of animation playing at waypoint that you are doing. [end shameless plug]
If you do it in the HB you need to check for !incoversation (and !incombat) to keep them from walking away while you are talking to them.